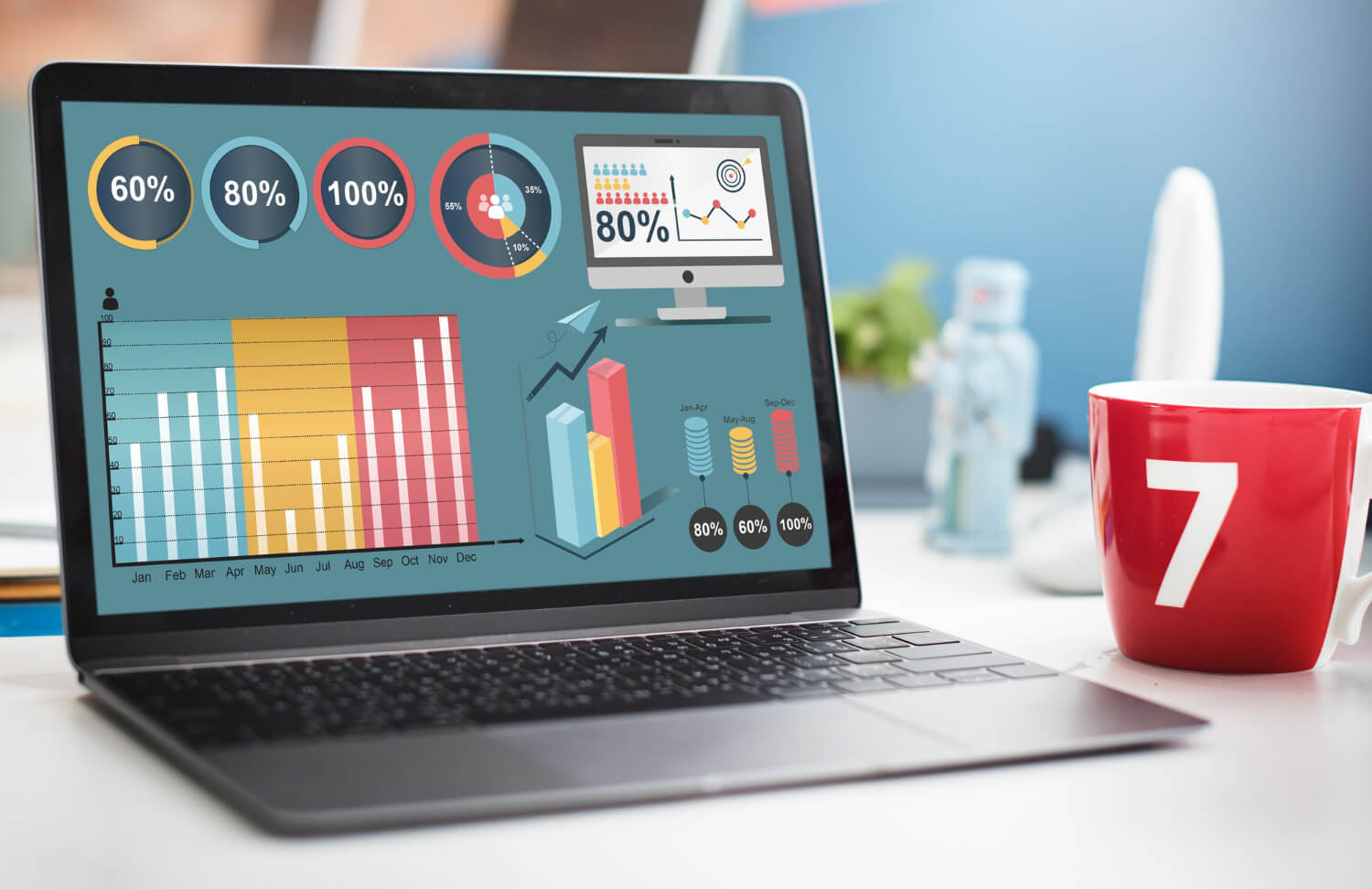
Last Updated | November 7, 2023
In Shopware 6, you can retrieve product data from the repository using various methods depending on your specific requirements and the context in which you’re working. Shopware 6 provides different ways to fetch product data, including basic repository methods, custom queries, and services. Here are some of the most common methods to get product data from the repository:
1. Using the Repository’s search Method:
The primary method for retrieving product data in Shopware 6 is the search method provided by the product repository. This method allows you to create complex queries to filter, sort, and paginate product data.
php
use ShopwareCoreContentProductProductCollection;
use ShopwareCoreContentProductProductDefinition;
$productRepository = $this->container->get(ProductDefinition::class)->getEntityRepository();
// Example: Retrieve all products
$criteria = new Criteria();
$products = $productRepository->search($criteria, Context::createDefaultContext());
You can customize the $criteria object to filter products based on various conditions, such as category, custom properties, price range, and more.
2. Using Services and Services Decorators:
Shopware 6 allows you to create custom services that interact with the repository to retrieve product data based on specific business logic. You can define these services in your custom plugins.
php
use ShopwareCoreContentProductSalesChannelProductSearchGatewayInterface;
class CustomProductService
{
private $productSearchGateway;
public function __construct(ProductSearchGatewayInterface $productSearchGateway)
{
$this->productSearchGateway = $productSearchGateway;
}
public function getProductsByCategory(string $categoryId)
{
$criteria = new Criteria();
$criteria->addFilter(new EqualsFilter(‘product.categories.id’, $categoryId));
return $this->productSearchGateway->search($criteria, Context::createDefaultContext());
}
}
You can create and use custom services to encapsulate specific queries for product data retrieval.
3. Using Custom Queries:
If you need to perform complex or custom queries on product data, you can write raw SQL queries using Shopware’s database connection service. While this approach is less recommended, it can be useful for specific use cases.
php
use ShopwareCoreFrameworkDataAbstractionLayerSearchCriteria;
$connection = $this->container->get(Connection::class);
$sql = ‘SELECT * FROM product WHERE …’;
$products = $connection->executeQuery($sql)->fetchAll();
Keep in mind that using custom SQL queries should be your last resort, as it bypasses the data abstraction layer and might lead to compatibility issues with future Shopware versions.
4. Using Repositories for Associated Entities:
In addition to the product repository, you can use repositories for associated entities like product reviews, product prices, or manufacturer information. These repositories allow you to fetch data related to products.
php
use ShopwareCoreContentProductReviewProductReviewRepository;
$productReviewRepository = $this->container->get(ProductReviewRepository::class);
$criteria = new Criteria();
$criteria->addFilter(new EqualsFilter(‘productId’, $productId));
$reviews = $productReviewRepository->search($criteria, Context::createDefaultContext());
Similarly, you can fetch data related to prices or manufacturers using their respective repositories.
These are some of the common methods to retrieve product data from the repository in Shopware 6. The choice of method depends on your specific use case and whether you need simple queries, custom logic, or direct access to associated entities. Always ensure that you follow best practices and consider the long-term maintainability of your code when working with repositories and services in Shopware 6.